Hi,
I'm having a really hard time rotating or translating assemblies. I have a bunch of step files and need to have them equally aligned. I'm loading the document with opencascade STEPCAFControl_Reader. To understand the overall principle I watched youtube (Quaoar - Lesson12&15) several times:
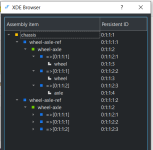
In simple cases where there is only one TopoDS_Solid as root node I do a BRepBuilderAPI_Transform on the shape and save it back. I'm not sure but I think this recalculates the whole geometry. This is not viable if the shape is referenced from multiple instances. The instance (wheelaxle-ref) has a TopLoc_Location attribute with its world-location in parents local location (child-parent-location is build up in traversal):
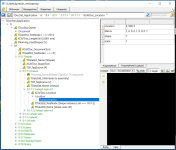
(Had to use TKInspector as AS asm-xde-dfbrowse is not showing Transformation)
As a example to understand how it could work I only try to change 1 wheelaxle-ref to see if it works!
In my project source I do the following to get the TopLoc_Location of TDF_Label after loading and writing it back to label:
This does work in viewer when running in lesson15_compose-XDE code as base but the exported STEP has no location showing same result. It seems only to work in direct viewer when running from memory. Also the Label gets a new name / tag:
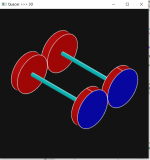
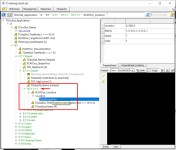
I also tried to rotate with using TopoDS_Shape:
But this never worked.
I did have a look at OpenCascade CAD-Builder. It does rotation but it seems to create a new rotated Shape and all Information like color and so on are missing. It even renames:
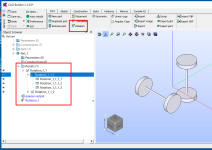
Now I'm totally confused. So these are my questions:
Please let me know if you need any more information for closer examination!!
Thank you very much! I very much appreciate the youtube videos of quaoar and your amount of work of Analysis Situs as I'm regularly having a peek into it's sources! Keep up the great work.
-Michael
I'm having a really hard time rotating or translating assemblies. I have a bunch of step files and need to have them equally aligned. I'm loading the document with opencascade STEPCAFControl_Reader. To understand the overall principle I watched youtube (Quaoar - Lesson12&15) several times:
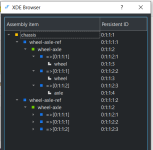
In simple cases where there is only one TopoDS_Solid as root node I do a BRepBuilderAPI_Transform on the shape and save it back. I'm not sure but I think this recalculates the whole geometry. This is not viable if the shape is referenced from multiple instances. The instance (wheelaxle-ref) has a TopLoc_Location attribute with its world-location in parents local location (child-parent-location is build up in traversal):
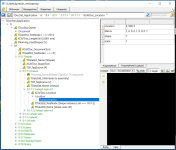
(Had to use TKInspector as AS asm-xde-dfbrowse is not showing Transformation)
As a example to understand how it could work I only try to change 1 wheelaxle-ref to see if it works!
In my project source I do the following to get the TopLoc_Location of TDF_Label after loading and writing it back to label:
C++:
void rotateByLabel(double angle, Handle(XCAFDoc_ShapeTool) aShapeTool) {
TDF_LabelSequence rootLabels;
aShapeTool->GetFreeShapes(rootLabels); // <-- retuns 1 chassis
for (int index = 1; index <= rootLabels.Length(); index++) {
for (TDF_ChildIterator itr(rootLabels.Value(index)); itr.More(); itr.Next()) { // has 2 items
TDF_Label label = itr.Value(); // returns wheelaxle-ref
gp_Trsf aTrsf = aShapeTool->GetLocation(label).Transformation();
// ... Do something with loc / transformation
aTrsf .SetTranslationPart(gp_Vec(0, 0, 50));
XCAFDoc_Location::Set(label, TopLoc_Location(aTrsf));
break; // Only one axle to see difference!
}
}
aShapeTool->UpdateAssemblies();
}
This does work in viewer when running in lesson15_compose-XDE code as base but the exported STEP has no location showing same result. It seems only to work in direct viewer when running from memory. Also the Label gets a new name / tag:
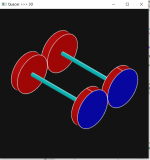
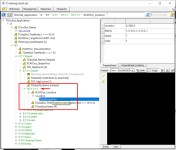
I also tried to rotate with using TopoDS_Shape:
C++:
void rotateByShape(double angle, Handle(XCAFDoc_ShapeTool) aShapeTool) {
TDF_LabelSequence rootLabels;
aShapeTool->GetFreeShapes(rootLabels); // <-- retuns 1 chassis
for (int index = 1; index <= rootLabels.Length(); index++) {
for (TDF_ChildIterator itr(rootLabels.Value(index)); itr.More(); itr.Next()) { // has 2 items
TDF_Label label = itr.Value(); // returns wheelaxle-ref
TopoDS_Shape shape = aShapeTool->GetShape(label);
gp_Trsf aTrsf = aShapeTool->GetLocation(label).Transformation();
// ... Do something with loc / transformation
aTrsf .SetTranslationPart(gp_Vec(0, 0, 50));
shape.Location(TopLoc_Location(aTrsf)); // Also tried shape.Move(aTrsf);
// Possibly set Shape back??
// aShapeTool->SetShape(label, shape);
break; // Only one axle to see difference!
}
}
aShapeTool->UpdateAssemblies();
}
I did have a look at OpenCascade CAD-Builder. It does rotation but it seems to create a new rotated Shape and all Information like color and so on are missing. It even renames:
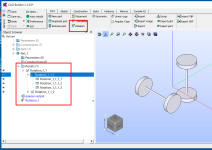
Now I'm totally confused. So these are my questions:
- Is it possible to rotate or translate a instance/assembly (not the referenced shape itsself) without copying?
- Or how to (re)set the location transformation to a new value?
- Can root-labels (e.g. FreeShapes) have a location?
Please let me know if you need any more information for closer examination!!
Thank you very much! I very much appreciate the youtube videos of quaoar and your amount of work of Analysis Situs as I'm regularly having a peek into it's sources! Keep up the great work.
-Michael
Last edited: