jianbaoxia
CAD master
Hi Bros, I'm JianBaoXia.
As title, I attempted to use BRepBndLib::AddOBB() to generate an OBB for four points that are not coplanar, but the results were confusing. Am I using it incorrectly?
There is my demo:
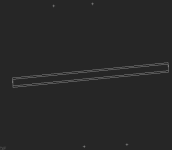
It is evident that this is an erroneous result, and I would greatly appreciate any assistance in resolving this issue.
As title, I attempted to use BRepBndLib::AddOBB() to generate an OBB for four points that are not coplanar, but the results were confusing. Am I using it incorrectly?
There is my demo:
Code:
bool WriteStepFile(const TopoDS_Shape& shape, const std::string& filepath)
{
bool output_flag = true;
STEPControl_Writer writer;
Interface_Static::SetCVal("write.step.schema", "AP203");
IFSelect_ReturnStatus status = writer.Transfer(shape, STEPControl_AsIs);
if (status != IFSelect_RetDone) output_flag = false;
status = writer.Write(filepath.c_str());
if (status != IFSelect_RetDone) output_flag = false;
return output_flag;
}
TopoDS_Shape GetEdges_of_obb(const Bnd_OBB& obb)
{
gp_Pnt centerPnt = obb.Center();
double xh = obb.XHSize(); // x_half_size
double yh = obb.YHSize();
double zh = obb.ZHSize();
//
gp_XYZ p0(xh, yh, zh);
gp_XYZ p1(-xh, yh, zh);
gp_XYZ p2(-xh, -yh, zh);
gp_XYZ p3(xh, -yh, zh);
gp_XYZ p4(xh, yh, -zh);
gp_XYZ p5(-xh, yh, -zh);
gp_XYZ p6(-xh, -yh, -zh);
gp_XYZ p7(xh, -yh, -zh);
//
gp_Pnt pnt0 = centerPnt.XYZ() + p0;
gp_Pnt pnt1 = centerPnt.XYZ() + p1;
gp_Pnt pnt2 = centerPnt.XYZ() + p2;
gp_Pnt pnt3 = centerPnt.XYZ() + p3;
gp_Pnt pnt4 = centerPnt.XYZ() + p4;
gp_Pnt pnt5 = centerPnt.XYZ() + p5;
gp_Pnt pnt6 = centerPnt.XYZ() + p6;
gp_Pnt pnt7 = centerPnt.XYZ() + p7;
//
TopoDS_Edge e01 = BRepBuilderAPI_MakeEdge(pnt0, pnt1);
TopoDS_Edge e12 = BRepBuilderAPI_MakeEdge(pnt1, pnt2);
TopoDS_Edge e23 = BRepBuilderAPI_MakeEdge(pnt2, pnt3);
TopoDS_Edge e30 = BRepBuilderAPI_MakeEdge(pnt3, pnt0);
TopoDS_Edge e45 = BRepBuilderAPI_MakeEdge(pnt4, pnt5);
TopoDS_Edge e56 = BRepBuilderAPI_MakeEdge(pnt5, pnt6);
TopoDS_Edge e67 = BRepBuilderAPI_MakeEdge(pnt6, pnt7);
TopoDS_Edge e74 = BRepBuilderAPI_MakeEdge(pnt7, pnt4);
TopoDS_Edge e04 = BRepBuilderAPI_MakeEdge(pnt0, pnt4);
TopoDS_Edge e15 = BRepBuilderAPI_MakeEdge(pnt1, pnt5);
TopoDS_Edge e26 = BRepBuilderAPI_MakeEdge(pnt2, pnt6);
TopoDS_Edge e37 = BRepBuilderAPI_MakeEdge(pnt3, pnt7);
//
TopoDS_Compound com;
BRep_Builder bb;
bb.MakeCompound(com);
bb.Add(com, e01);
bb.Add(com, e12);
bb.Add(com, e23);
bb.Add(com, e30);
bb.Add(com, e45);
bb.Add(com, e56);
bb.Add(com, e67);
bb.Add(com, e74);
bb.Add(com, e04);
bb.Add(com, e15);
bb.Add(com, e26);
bb.Add(com, e37);
return com;
}
int main()
{
gp_Pnt p0(-124.989, 36.9042, 30);
gp_Pnt p1(-130.293, 40.0692, 0);
gp_Pnt p2(-127.948, 48.6445, 0);
gp_Pnt p3(-123.193, 44.6424, 30);
TopoDS_Compound com1;
BRep_Builder bb1;
bb1.MakeCompound(com1);
bb1.Add(com1, BRepBuilderAPI_MakeVertex(p0));
bb1.Add(com1, BRepBuilderAPI_MakeVertex(p1));
bb1.Add(com1, BRepBuilderAPI_MakeVertex(p2));
bb1.Add(com1, BRepBuilderAPI_MakeVertex(p3));
Bnd_OBB obb;
BRepBndLib::AddOBB(com1, obb);
TopoDS_Shape obb_wireFrame = GetEdges_of_obb(obb);
TopoDS_Compound com;
BRep_Builder bb;
bb.MakeCompound(com);
bb.Add(com, obb_wireFrame);
bb.Add(com, BRepBuilderAPI_MakeVertex(p0));
bb.Add(com, BRepBuilderAPI_MakeVertex(p1));
bb.Add(com, BRepBuilderAPI_MakeVertex(p2));
bb.Add(com, BRepBuilderAPI_MakeVertex(p3));
// bb.Add(com, ruleFace_sec);
// bb.Add(com, ruledFace);
WriteStepFile(com, "../obb.step");
return 0;
}
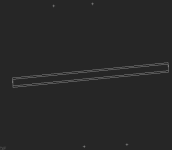
It is evident that this is an erroneous result, and I would greatly appreciate any assistance in resolving this issue.